똑 같은 내용으로 파이썬 프로그래밍과 소스코드는 이곳을 참조한다.
http://www.circuitbasics.com/raspberry-pi-lcd-set-up-and-programming-in-python
Wiring the LCD in 8 Bit Mode 연결
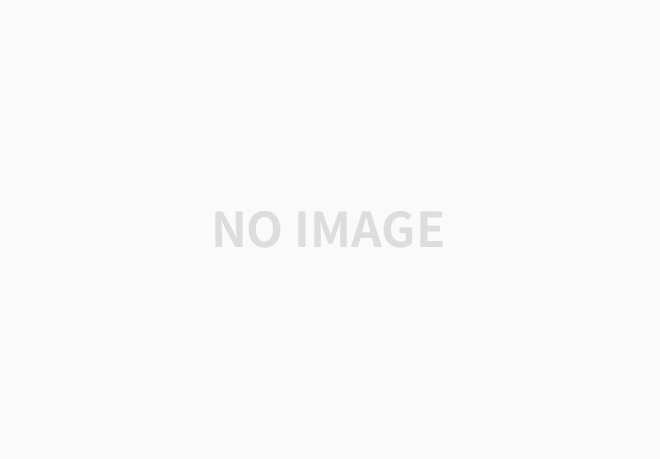
Wiring the LCD in 4 Bit Mode 연결
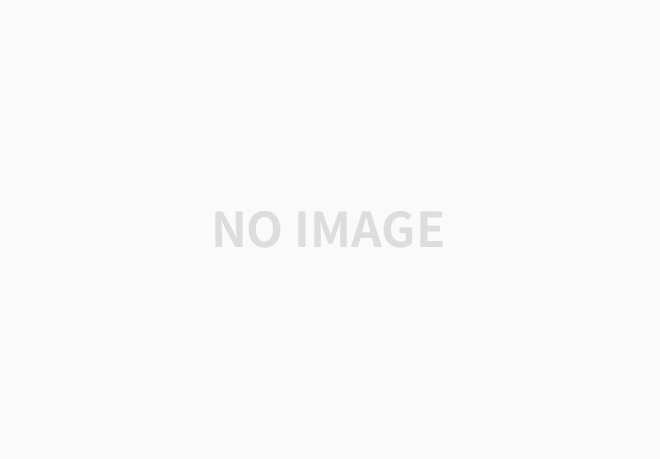
위 연결도의 밝기와 선명도 조절 가변저항은 10K, 저항이나 1K~3K 저항을 사용하면 된다.
wiringPi 설치
C언어를 사용하여 라즈베리 파이를 프로그래밍 하기 위해 wiringPi 라이브러리를 설치한다. 이미 설치되어 있다면 건너 뛴다.
아래 명령어를 사용하여 wiringPi 를 다운받아 컴파일한다.
1. Git 을 사용하여 WiringPi 를 설치할 수 있는 git-core 설치
$sudo apt-get install git-core
만약 에러가 난다면 다음 명령어를 사용하여 업데이트를 한다.
$sudo apt-get update
2. WiringPi 를 다운로드
$git clone git://git.drogon.net/wiringPi
3. 체인지 디렉토리 명령
$cd wiringPi
4. 설치 명령을 입력
$./build
5. 설치가 잘 되었는지 아래 명령어로 확인
$gpio -v
$gpio readall
C로 만들어진 LCD 제어 프로그램의 컴파일과 실행방법 - 자신의 프로그램이 example.c 라 하면
컴파일
$gcc -o example example.c -lwiringPi -lwiringPiDev
실행
$sudo ./example
Write to Display in 8 Bit Mode
LCD 화면에 "Hello, World!" 하고 표시한다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
|
#include <wiringPi.h>
#include <lcd.h>
//USE WIRINGPI PIN NUMBERS
#define LCD_RS 25 //Register select pin
#define LCD_E 24 //Enable Pin
#define LCD_D0 29 //Data pin D0
#define LCD_D1 28 //Data pin D1
#define LCD_D2 27 //Data pin D2
#define LCD_D3 26 //Data pin D3
#define LCD_D4 23 //Data pin D4
#define LCD_D5 22 //Data pin D5
#define LCD_D6 21 //Data pin D6
#define LCD_D7 14 //Data pin D7
int main()
{
int lcd;
wiringPiSetup();
lcd = lcdInit (2, 16, 8, LCD_RS, LCD_E, LCD_D0, LCD_D1, LCD_D2, LCD_D3, LCD_D4, LCD_D5, LCD_D6, LCD_D7);
lcdPuts(lcd, "Hello, world!");
}
|
cs |
lcd = lcdInit (ROWS, COLUMNS, BIT MODE, LCD_RS, LCD_E, LCD_D0, LCD_D1, LCD_D2, LCD_D3, LCD_D4, LCD_D5, LCD_D6, LCD_D7);
이런 인자들로 구성됨을 확인한다.
Write to Display in 4 Bit Mode
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
|
#include <wiringPi.h>
#include <lcd.h>
//USE WIRINGPI PIN NUMBERS
#define LCD_RS 25 //Register select pin
#define LCD_E 24 //Enable Pin
#define LCD_D4 23 //Data pin 4
#define LCD_D5 22 //Data pin 5
#define LCD_D6 21 //Data pin 6
#define LCD_D7 14 //Data pin 7
int main()
{
int lcd;
wiringPiSetup();
lcd = lcdInit (2, 16, 4, LCD_RS, LCD_E, LCD_D4, LCD_D5, LCD_D6, LCD_D7, 0, 0, 0, 0);
lcdPuts(lcd, "Hello, world!");
}
|
cs |
Position the Text
LCD 에서 위치 변경을 위해 lcdPosition(lcd, COLUMN, ROW) 함수를 사용한다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
|
#include <wiringPi.h>
#include <lcd.h>
//USE WIRINGPI PIN NUMBERS
#define LCD_RS 25 //Register select pin
#define LCD_E 24 //Enable Pin
#define LCD_D4 23 //Data pin 4
#define LCD_D5 22 //Data pin 5
#define LCD_D6 21 //Data pin 6
#define LCD_D7 14 //Data pin 7
int main()
{
int lcd;
wiringPiSetup();
lcd = lcdInit (2, 16, 4, LCD_RS, LCD_E, LCD_D4, LCD_D5, LCD_D6, LCD_D7, 0, 0, 0, 0);
lcdPosition(lcd, 3, 1);
lcdPuts(lcd, "Hello, world!");
}
|
cs |
Clear the Screen
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
|
#include <wiringPi.h>
#include <lcd.h>
//USE WIRINGPI PIN NUMBERS
#define LCD_RS 25 //Register select pin
#define LCD_E 24 //Enable Pin
#define LCD_D4 23 //Data pin 4
#define LCD_D5 22 //Data pin 5
#define LCD_D6 21 //Data pin 6
#define LCD_D7 14 //Data pin 7
int main()
{
int lcd;
wiringPiSetup();
lcd = lcdInit (2, 16, 4, LCD_RS, LCD_E, LCD_D4, LCD_D5, LCD_D6, LCD_D7, 0, 0, 0, 0);
lcdPuts(lcd, "This is how you");
sleep(2);
lcdClear(lcd);
lcdPuts(lcd, "clear the screen");
sleep(2);
lcdClear(lcd);
}
|
cs |
Blinking Text
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
|
#include <wiringPi.h>
#include <lcd.h>
//USE WIRINGPI PIN NUMBERS
#define LCD_RS 25 //Register select pin
#define LCD_E 24 //Enable Pin
#define LCD_D4 23 //Data pin 4
#define LCD_D5 22 //Data pin 5
#define LCD_D6 21 //Data pin 6
#define LCD_D7 14 //Data pin 7
int main()
{
int lcd;
wiringPiSetup();
lcd = lcdInit (2, 16, 4, LCD_RS, LCD_E, LCD_D4, LCD_D5, LCD_D6, LCD_D7, 0, 0, 0, 0);
while(1){
lcdPosition(lcd, 0, 0);
lcdPuts(lcd, "Hello, world!");
sleep(2);
lcdClear(lcd);
sleep(2);
}
}
|
cs |
Cursor On/Off
- Underline non-blinking cursor: lcdCursor(lcd, 1)
- Underline blinking cursor: lcdCursor(lcd, 1), followed by lcdCursorBlink(lcd, 1)
- Blinking block style cursor: lcdCursorBlink(lcd, 1)
- Cursor off : lcdCursor(lcd, 0)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
|
#include <wiringPi.h>
#include <lcd.h>
//USE WIRINGPI PIN NUMBERS
#define LCD_RS 25 //Register select pin
#define LCD_E 24 //Enable Pin
#define LCD_D4 23 //Data pin 4
#define LCD_D5 22 //Data pin 5
#define LCD_D6 21 //Data pin 6
#define LCD_D7 14 //Data pin 7
int main()
{
int lcd;
wiringPiSetup();
lcd = lcdInit (2, 16, 4, LCD_RS, LCD_E, LCD_D4, LCD_D5, LCD_D6, LCD_D7, 0, 0, 0, 0);
//lcdCursor(lcd, 0); //Cursor OFF
lcdCursor(lcd, 1); //Cursor ON, underline, not blinking
//lcdCursorBlink(lcd, 1); //Cursor ON, block, blinking
//Use both lines below to get a blinking underline/block cursor
//lcdCursor(lcd, 1);
//lcdCursorBlink(lcd, 1);
lcdPuts(lcd, "Hello, world!");
}
|
cs |
Print the Date and Time
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
|
#include <wiringPi.h>
#include <lcd.h>
#include <stdio.h>
#include <time.h>
//USE WIRINGPI PIN NUMBERS
#define LCD_RS 25 //Register select pin
#define LCD_E 24 //Enable Pin
#define LCD_D4 23 //Data pin 4
#define LCD_D5 22 //Data pin 5
#define LCD_D6 21 //Data pin 6
#define LCD_D7 14 //Data pin 7
int main()
{
int lcd;
wiringPiSetup();
lcd = lcdInit (2, 16, 4, LCD_RS, LCD_E, LCD_D4, LCD_D5, LCD_D6, LCD_D7, 0, 0, 0, 0);
while(1){
time_t timer;
char buffer_date[26];
char buffer_time[26];
struct tm* tm_info;
time(&timer);
tm_info = localtime(&timer);
strftime(buffer_date, 26, "Date: %m:%d:%Y", tm_info);
strftime(buffer_time, 26, "Time: %H:%M:%S", tm_info);
lcdPosition(lcd, 0, 0);
lcdPuts(lcd, buffer_date);
lcdPosition(lcd, 0, 1);
lcdPuts(lcd, buffer_time);
}
}
|
cs |
Print Your IP Address
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
|
#include <wiringPi.h>
#include <lcd.h>
#include <stdio.h>
#include <string.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <sys/ioctl.h>
#include <netinet/in.h>
#include <net/if.h>
#include <unistd.h>
#include <arpa/inet.h>
//USE WIRINGPI PIN NUMBERS
#define LCD_RS 25 //Register select pin
#define LCD_E 24 //Enable Pin
#define LCD_D4 23 //Data pin 4
#define LCD_D5 22 //Data pin 5
#define LCD_D6 21 //Data pin 6
#define LCD_D7 14 //Data pin 7
int main()
{
int lcd;
wiringPiSetup();
lcd = lcdInit (2, 16, 4, LCD_RS, LCD_E, LCD_D4, LCD_D5, LCD_D6, LCD_D7, 0, 0, 0, 0);
int n;
struct ifreq ifr;
char iface[] = "eth0"; //Change this to the network of your choice (eth0, wlan0, etc.)
n = socket(AF_INET, SOCK_DGRAM, 0);
ifr.ifr_addr.sa_family = AF_INET;
strncpy(ifr.ifr_name , iface , IFNAMSIZ - 1);
ioctl(n, SIOCGIFADDR, &ifr);
close(n);
lcdPosition(lcd, 0, 0);
lcdPrintf(lcd, "IP Address: ");
lcdPosition(lcd, 0, 1);
lcdPrintf(lcd, ("%s - %s\n" , iface , inet_ntoa(( (struct sockaddr_in *)&ifr.ifr_addr )->sin_addr)));
return 0;
}
|
cs |
Custom Characters
Printing a Single Custom Character
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
|
#include <wiringPi.h>
#include <lcd.h>
//USE WIRINGPI PIN NUMBERS
#define LCD_RS 25 //Register select pin
#define LCD_E 24 //Enable Pin
#define LCD_D4 23 //Data pin 4
#define LCD_D5 22 //Data pin 5
#define LCD_D6 21 //Data pin 6
#define LCD_D7 14 //Data pin 7
char omega[8] = { 0b00000,
0b01110,
0b10001,
0b10001,
0b10001,
0b01010,
0b11011,
0b00000};
void customChar(void);
int lcd;
int main()
{
int lcd;
wiringPiSetup();
lcd = lcdInit (2, 16, 4, LCD_RS, LCD_E, LCD_D4, LCD_D5, LCD_D6, LCD_D7, 0, 0, 0, 0);
customChar();
}
void customChar(void)
{
lcdCharDef(lcd, 2, omega);
lcdClear(lcd);
lcdPutchar(lcd, 2);
sleep(3);
}
|
cs |
Printing Multiple Custom Characters
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
|
#include <wiringPi.h>
#include <lcd.h>
//USE WIRINGPI PIN NUMBERS
#define LCD_RS 25 //Register select pin
#define LCD_E 24 //Enable Pin
#define LCD_D4 23 //Data pin 4
#define LCD_D5 22 //Data pin 5
#define LCD_D6 21 //Data pin 6
#define LCD_D7 14 //Data pin 7
char omega[8] = { 0b00000,
0b01110,
0b10001,
0b10001,
0b10001,
0b01010,
0b11011,
0b00000};
char pi[8] = { 0b00000,
0b00000,
0b11111,
0b01010,
0b01010,
0b01010,
0b10011,
0b00000};
char mu[8] = { 0b00000,
0b10010,
0b10010,
0b10010,
0b10010,
0b11101,
0b10000,
0b10000};
char drop[8] = { 0b00100,
0b00100,
0b01010,
0b01010,
0b10001,
0b10001,
0b10001,
0b01110};
char temp[8] = { 0b00100,
0b01010,
0b01010,
0b01110,
0b01110,
0b11111,
0b11111,
0b01110};
void customChar(void);
int lcd;
int main()
{
int lcd;
wiringPiSetup();
lcd = lcdInit (2, 16, 4, LCD_RS, LCD_E, LCD_D4, LCD_D5, LCD_D6, LCD_D7, 0, 0, 0, 0);
customChar();
}
void customChar(void)
{
lcdCharDef(lcd, 10, omega);
lcdCharDef(lcd, 11, pi);
lcdCharDef(lcd, 12, mu);
lcdCharDef(lcd, 13, drop);
lcdCharDef(lcd, 14, temp);
lcdClear(lcd);
lcdPutchar(lcd, 10);
lcdPutchar(lcd, 11);
lcdPutchar(lcd, 12);
lcdPutchar(lcd, 13);
lcdPutchar(lcd, 14);
sleep(3);
}
|
cs |
Scrolling Text
Scroll Right to Left
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
|
#include <stdio.h>
#include <wiringPi.h>
#include <lcd.h>
#include <string.h>
//USE WIRINGPI PIN NUMBERS
#define LCD_RS 25 //Register select pin
#define LCD_E 24 //Enable Pin
#define LCD_D4 23 //Data pin 4
#define LCD_D5 22 //Data pin 5
#define LCD_D6 21 //Data pin 6
#define LCD_D7 14 //Data pin 7
void scrollText(void);
char message[] = "Hello, world!";
int count = 0;
int j = 0;
int lcd;
int main()
{
wiringPiSetup();
lcd = lcdInit (2, 16, 4, LCD_RS, LCD_E, LCD_D4, LCD_D5, LCD_D6, LCD_D7, 0, 0, 0, 0);
while(1){
scrollText();
}
}
void scrollText(void)
{
int i, n;
int h;
int tempSpace = 0;
char scrollPadding[] = " ";
int messageLength = strlen(scrollPadding) + strlen(message);
for (n = 0; n < messageLength; n++){h = 16; usleep(300000); printf("\x1B[2J"); if (j > messageLength)
j = 0;
for (i = 0; i < j ; i++){
scrollPadding[h - j] = message[i];
h++;
}
lcdPosition(lcd, 0, 0);
lcdClear(lcd);
lcdPrintf(lcd, "%s", scrollPadding);
j++;
}
}
|
cs |
Scroll Left to Right
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
|
#include <stdio.h>
#include <wiringPi.h>
#include <lcd.h>
#include <string.h>
//USE WIRINGPI PIN NUMBERS
#define LCD_RS 25 //Register select pin
#define LCD_E 24 //Enable Pin
#define LCD_D4 23 //Data pin 4
#define LCD_D5 22 //Data pin 5
#define LCD_D6 21 //Data pin 6
#define LCD_D7 14 //Data pin 7
void scrollText(void);
char message[] = "Hello, world!";
int count = 0;
int j = 0;
int lcd;
int main()
{
wiringPiSetup();
lcd = lcdInit (2, 16, 4, LCD_RS, LCD_E, LCD_D4, LCD_D5, LCD_D6, LCD_D7, 0, 0, 0, 0);
while(1){
scrollText();
}
}
void scrollText(void)
{
int i, n;
int h;
int tempSpace = 0;
char scrollPadding[] = " ";
int messageLength = strlen(scrollPadding) + strlen(message);
for (n = 0; n < messageLength; n++){h = 16; usleep(300000); printf("\x1B[2J"); if (j > messageLength)
j = 0;
for (i = strlen(message); i >= 0; i--){
scrollPadding[j - h] = message[i];
h++;
}
lcdPosition(lcd, 0, 0);
lcdClear(lcd);
lcdPrintf(lcd, "%s", scrollPadding);
j++;
}
}
|
cs |
Print Data From a Sensor
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
|
#include <wiringPi.h>
#include <lcd.h>
#include <stdio.h>
#include <stdlib.h>
#include <stdint.h>
//USE WIRINGPI PIN NUMBERS
#define LCD_RS 25 //Register select pin
#define LCD_E 24 //Enable Pin
#define LCD_D4 23 //Data pin 4
#define LCD_D5 22 //Data pin 5
#define LCD_D6 21 //Data pin 6
#define LCD_D7 14 //Data pin 7
#define MAXTIMINGS 85
#define DHTPIN 7
int lcd;
int dht11_dat[5] = {0, 0, 0, 0, 0};
void read_dht11_dat()
{
uint8_t laststate = HIGH;
uint8_t counter = 0;
uint8_t j = 0, i;
float f;
dht11_dat[0] = dht11_dat[1] = dht11_dat[2] = dht11_dat[3] = dht11_dat[4] = 0;
pinMode(DHTPIN, OUTPUT);
digitalWrite(DHTPIN, LOW);
delay(18);
digitalWrite(DHTPIN, HIGH);
delayMicroseconds(40);
pinMode(DHTPIN, INPUT);
for (i = 0; i < MAXTIMINGS; i++)
{
counter = 0;
while (digitalRead(DHTPIN) == laststate)
{
counter++;
delayMicroseconds(1);
if (counter == 255)
{
break;
}
}
laststate = digitalRead(DHTPIN);
if (counter == 255)
break;
if ((i >= 4) && (i % 2 == 0))
{
dht11_dat[j / 8] <<= 1;
if (counter > 16)
dht11_dat[j / 8] |= 1;
j++;
}
}
if ((j >= 40) && (dht11_dat[4] == ((dht11_dat[0] + dht11_dat[1] + dht11_dat[2] + dht11_dat[3]) & 0xFF)))
{
f = dht11_dat[2] * 9. / 5. + 32;
lcdPosition(lcd, 0, 0);
lcdPrintf(lcd, "Humidity: %d.%d %%\n", dht11_dat[0], dht11_dat[1]);
lcdPosition(lcd, 0, 1);
//lcdPrintf(lcd, "Temp: %d.0 C", dht11_dat[2]); //Uncomment for Celcuis
lcdPrintf(lcd, "Temp: %.1f F", f); //Comment out for Celcuis
}
}
int main(void)
{
int lcd;
wiringPiSetup();
lcd = lcdInit (2, 16, 4, LCD_RS, LCD_E, LCD_D4, LCD_D5, LCD_D6, LCD_D7, 0, 0, 0, 0);
while (1)
{
read_dht11_dat();
delay(1000);
}
return(0);
}
|
cs |
더욱 좋은 정보를 제공하겠습니다.~ ^^
'개발자 > Raspberry Pi' 카테고리의 다른 글
wiringPi GPIO 설정 이렇게 초기화 된다. (0) | 2017.03.26 |
---|---|
Rapsberry Pi 3 Access Point 만들기 AP 만들기 (0) | 2017.03.23 |
라즈베리 파이에서 node js 로 온습도 센서 dht22에서 데이터 읽어오기 (4) | 2017.03.14 |
라즈베리파이와 MCP3208 ADC 컨버터 사용하기 - 회로와 소스코드 (30) | 2017.02.12 |
라즈베리 파이 Serial 프로그램 예제와 설명 (0) | 2017.02.06 |
1월 25일 K-ICT 디바이스랩 판교에서 삼성 ARTIK 소개 (0) | 2017.01.26 |
라즈베리파이 공식 7인치 터치스크린 (Raspberry-Pi Touch Display) (Rev 1.1) (0) | 2017.01.17 |
라즈베리 파이 LoRa 통신 모듈 테스트 남은 일들 (2) | 2016.12.20 |